C language address book management system_address book management system (C language)
#include<stdio.h>
#include<stdlib.h> //The header file of dynamic memory allocation malloc
#include<conio.h>
#include<dos.h>
#include<string.h>
#define LEN sizeof(struct Link) //symbolic constant whose number of bytes is the number of bytes of struct Link
struct Link //Define the contact information structure
{
char name[20]; //name
char unit[20]; //Work unit
char phone[15]; //phone number
char mail[20]; //E-mail address
}Link_s[100]; //Can store 100 contact information
//declare the functions to be used in the program
void input(); //Create new contact information
void enquiry(); //Query contact information
void alter(); //modify contact information
void del(); //delete contact information
void display(); //Display contact information
void menu(); //Main menu
void menu()//Main menu function
{
system("cls");//Call the system("cls") function to clear the interface
printf("nnnnn");//Control the display position of the main function menu
printf("tt|***************Address management system***************|n");
printf("tt| 1. New contact information|n");
printf("tt| 2. Query contact information|n");
printf("tt| 3. Modify contact information|n");
printf("tt| 4. Delete contact information|n");
printf("tt| 5. Display contact information|n");
printf("tt| 6. Exit the system|n");
printf("tt|********************************************|n");
printf("Please select function (1~6): n");
}
int main()//main function
{
int n;
menu();//Call the main menu
scanf("%d", &n);//User selection function
while(n)
{
switch (n)
{
case 1:input(); break;
case 2:enquiry(); break;
case 3:alter(); break;
case 4:del(); break;
case 5:display(); break;
case 6:exit(0);
}
printf("Please select the function (1~6): ");
scanf("%d", &n);//User selection function
}
return 0;
}
void input() //Create a new contact
{
int i, record = 0;//Define a loop variable and a variable that records the number of contacts in the address book
char ch[2];//The character array is used to record the user's input
FILE* fp;//Define the pointer variable to the file
if ((fp=fopen("data.txt", "a+")) == NULL)//Call the fopen function to create a new text to save the contact information
{
printf("Can't open folder!n");
return;
}
while (feof(fp) == 0)//Determine whether the file is over
{
if (fread(&Link_s[record], LEN, 1, fp) == 1)//Use the fresd function to read the records in the file into the structure array one by one
record++;
}
fclose(fp);//Close the file
if (record == 0)//Determine whether the current address book is empty
printf("No contact record!n");
else
{
system("cls");//Clear the screen
display();//Display all the information of the contact
}
if ((fp = fopen("data.txt", "wb")) == NULL)//If opening the file fails
{
printf("Cannot open the file!n");
return;
}
//rewrite data to disk
for (i = 0; i < record; i++)
fwrite(&Link_s[i], LEN, 1, fp);
printf("Whether to create a new contact (y/n)");//Prompt the user for input
scanf("%s", ch);
while (strcmp(ch, "Y") == 0 || strcmp(ch, "y") == 0)//Determine whether the user needs to create a new one
{
printf("Name:");
scanf("%s", &Link_s[record].name);
/ / Determine whether the user input name exists in the address book
for (i = 0; i < record; i++)
if (strcmp(Link_s[i].name, Link_s[record].name) == 0)
{
printf("Name already exists, please press any key to continue.");
getch();
fclose(fp);
return;
}
printf("Work unit:");
scanf("%s", &Link_s[record].unit);
printf("Phone number:");
scanf("%s", &Link_s[record].phone);
printf("E-mail:");
scanf("%s", &Link_s[record].mail);
if (fwrite(&Link_s[record], LEN, 1, fp) != 1)//Save the new contact information to a disk file
{
printf("Cannot save record!");
getch(); //Press any key to continue
}
else
{
printf("%s information saved successfully!n", Link_s[record].name);
record++;
}
printf("Continue to create new contacts? (y/n):");
scanf("%s", ch);
}
fclose(fp);
printf("New completion!n");
}
void enquiry() //Query contacts
{
menu();//Call the main menu
FILE* fp;
int i, n, record = 0;
char ch[2], name[20], phone[15];//Define the array to receive the user's input information
//Open the disk file and read the address book in the disk file to the memory structure array one by one
if ((fp = fopen("data.txt", "rb")) == NULL)
{
printf("Cannot open the file n");
return;
}
while (feof(fp) == 0)//Determine whether the end of the file is reached
if (fread(&Link_s[record], LEN, 1, fp) == 1)
record++;//The final value of record is the address book entry
fclose(fp);
if (record == 0)
{
printf("No contact info! n");
return;
}
printf("Search by name, enter 1, search by phone number, enter 2: ");
scanf("%d", &n);
if (n == 1)
{
printf("Please enter your name:");
scanf("%s", name);
}
if (n == 2)
{
printf("Please enter the phone number:");
scanf("%s", phone);
}
/ / Find the specified information in the address book by name or phone number, and use the character comparison function
for (i = 0; i < record; i++)
if ((strcmp(name, Link_s[i].name) == 0) || (strcmp(phone, Link_s[i].phone) == 0))
{
printf("Find the contact, whether to display it? (y/n):");
scanf("%s", ch);
if (strcmp(ch, "Y") == 0 || strcmp(ch, "y") == 0)
{
printf("Name work unit phone number E-mail tn ");
printf("%-s %-s %-s %-sn", Link_s[i].name, Link_s[i].unit, Link_s[i].phone, Link_s[i].mail);
}
break;
}
/ / Determine whether the loop ends because the contact is not found or because it is found but the contact information is not displayed
if (i == record)
printf("No contact found! n");
}
void alter() //modify contact information
{
menu();//Call the main menu
FILE* fp;
int i, j, record = 0;
char name[20];
if ((fp = fopen("data.txt", "r+")) == NULL)
{
printf("Cannot open file!n");
return;
}
while (feof(fp) == 0)
if (fread(&Link_s[record], LEN, 1, fp) == 1)
record++;
if (record == 0)
{
printf("No contact record!n");
fclose(fp);
return;
}
display();
//Modify contact information//
printf("Please enter the name of the contact you want to modify: n");
scanf("%s", &name);
for (i = 0; i < record; i++)
{
if (strcmp(name, Link_s[i].name) == 0)
{
printf("Find the contact! You can modify the data! n");
printf("Name:");
scanf("%s", &Link_s[i].name);
printf("Work unit:");
scanf("%s", &Link_s[i].unit);
printf("Phone number:");
scanf("%s", &Link_s[i].phone);
printf("E-mail address:");
scanf("%s", &Link_s[i].mail);
printf("Modified successfully!");
//Open the disk and write the modified contact information to the disk
if ((fp = fopen("data.txt", "wb")) == NULL)
{
printf("Can't open file! n");
return;
}
for (j = 0; j < record; j++)
if (fwrite(&Link_s[j], LEN, 1, fp) != 1)
{
printf("Cannot save!");
getch(); //Press any key to continue
}
fclose(fp);
return;
}
}
printf("The contact information was not found! n");//The contact information was not found
}
void del() //delete contact information
{
menu();//Call the main menu
FILE* fp;
int i, j, record = 0;
char ch[2];
char name[15];
if ((fp = fopen("data.txt", "r+")) == NULL)
{
printf("Cannot open the file!n");
return;
}
while (feof(fp) == 0)
if (fread(&Link_s[record], LEN, 1, fp) == 1)
record++;
fclose(fp);
if (record == 0)
{
printf("No such contact record!n");
return;
}
display();
// delete contact information
printf("Please enter the name of the contact you want to delete: ");
scanf("%s", &name);
for (i = 0; i < record; i++)
{
if (strcmp(name, Link_s[i].name) == 0)
{
printf("Found this contact, do you want to delete it?(y/n)");
scanf("%s", ch);
if (strcmp(ch, "Y") == 0 || strcmp(ch, "y") == 0)
for (j = i; j < record; j++)
Link_s[j] = Link_s[j + 1];
record--;
//Write the deleted address book to the corresponding disk file
if ((fp = fopen("data.txt", "wb")) == NULL)
{
printf("Cannot open file!n");
return;
}
for (j = 0; j < record; j++)
if (fwrite(&Link_s[j], LEN, 1, fp) != 1)
{
printf("Cannot save!n");
getch(); //Press any key to continue
}
fclose(fp);
printf("Deleted successfully!n");
return;
}
}
printf("No such contact record!n");
}
void display() //Display contact information
{
menu();//Call the main menu
FILE* fp;
int i, record = 0;
fp = fopen("data.txt", "rb");//Open the file for reading and writing
//Read the address book record
while (feof(fp) == 0)
{
if (fread(&Link_s[record], LEN, 1, fp) == 1)
record++;
}
fclose(fp);//Close the file
printf("Name work unit phone number E-mail tn");
for (i = 0; i < record; i++)
printf("%-s %-s
%-s %-sn", Link_s[i].name, Link_s[i].unit, Link_s[i].phone, Link_s[i].mail);
}
run screenshot
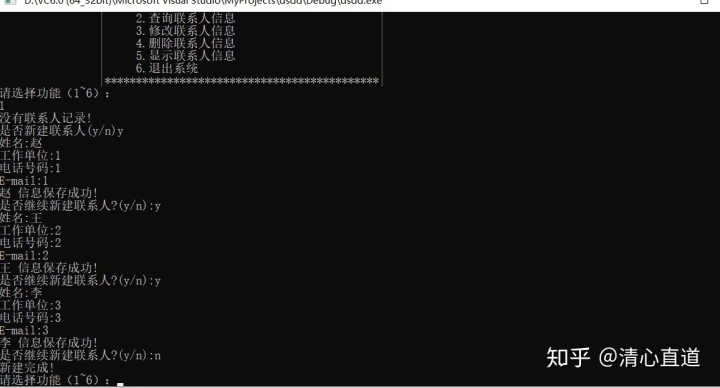
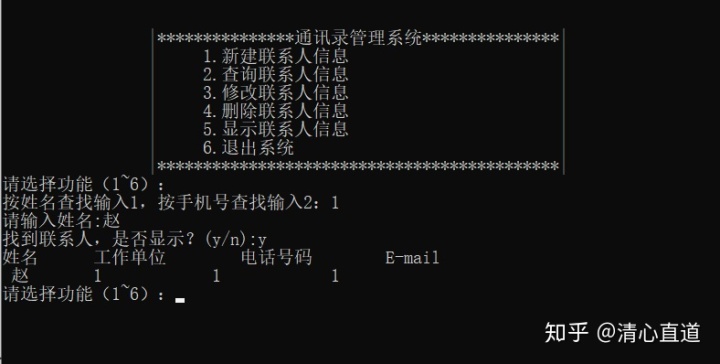
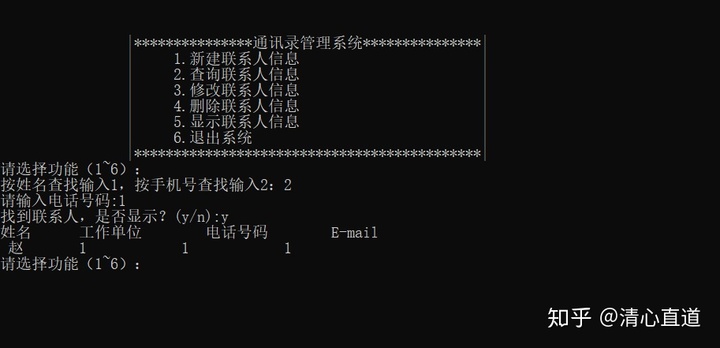
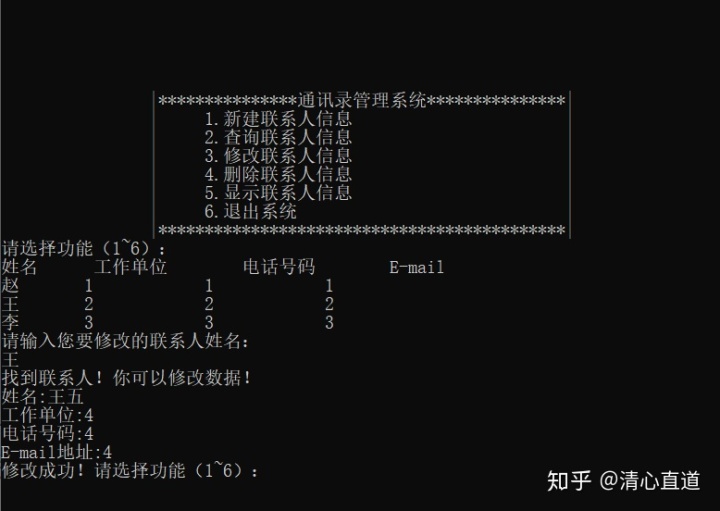
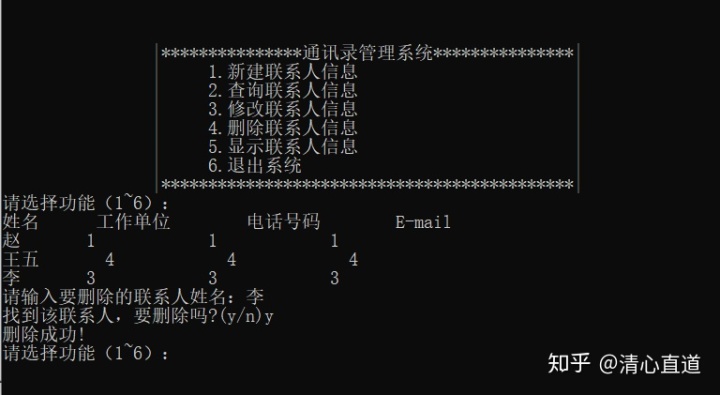
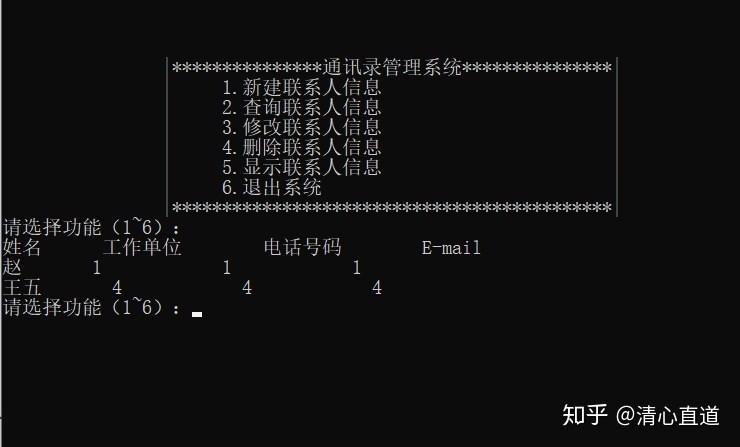